# | Problem | Pass Rate (passed user / total user) |
---|---|---|
11459 | Cheat Sheet |
|
12261 | Matrix |
|
13116 | ReadFirst for I2P2 Mid2 Exam |
|
13910 | Branny's naming conventions (1/3) |
|
13911 | Branny's naming conventions (2/3) |
|
13912 | Branny's naming conventions (3/3) |
|
13914 | Soccer (1/3) |
|
13915 | Soccer (2/3) |
|
13916 | Soccer (3/3) |
|
Description
Array.cpp // overloaded assignment operator; // const return avoids: ( a1 = a2 ) = a3 const Array &Array::operator=( const Array &right ) { if ( &right != this ) // avoid self-assignment { // for Arrays of different sizes, deallocate original // left-side Array, then allocate new left-side Array if ( size != right.size ) { delete [] ptr; // release space size = right.size; // resize this object ptr = new int[ size ]; // create space for Array copy } // end inner if for ( size_t i = 0; i < size; ++i ) ptr[ i ] = right.ptr[ i ]; // copy array into object } // end outer if return *this; // enables x = y = z, for example } // end function operator= // determine if two Arrays are equal and // return true, otherwise return false bool Array::operator==( const Array &right ) const { if ( size != right.size ) return false; // arrays of different number of elements for ( size_t i = 0; i < size; ++i ) if ( ptr[ i ] != right.ptr[ i ] ) return false; // Array contents are not equal return true; // Arrays are equal } // end function operator== // overloaded subscript operator for non-const Arrays; // reference return creates a modifiable lvalue int &Array::operator[]( int subscript ) { // check for subscript out-of-range error if ( subscript < 0 || subscript >= size ) throw out_of_range( "Subscript out of range" ); return ptr[ subscript ]; // reference return } // end function operator[] // overloaded subscript operator for const Arrays // const reference return creates an rvalue int Array::operator[]( int subscript ) const { // check for subscript out-of-range error if ( subscript < 0 || subscript >= size ) throw out_of_range( "Subscript out of range" ); return ptr[ subscript ]; // returns copy of this element } // end function operator[] // overloaded input operator for class Array; // inputs values for entire Array istream &operator>>( istream &input, Array &a ) { for ( size_t i = 0; i < a.size; ++i ) input >> a.ptr[ i ]; return input; // enables cin >> x >> y; } // end function // overloaded output operator for class Array ostream &operator<<( ostream &output, const Array &a ) { // output private ptr-based array for ( size_t i = 0; i < a.size; ++i ) { output << setw( 12 ) << a.ptr[ i ]; if ( ( i + 1 ) % 4 == 0 ) // 4 numbers per row of output output << endl; } // end for if ( a.size % 4 != 0 ) // end last line of output output << endl; return output; // enables cout << x << y; } // end function operator<<
Inheritance type |
Public |
Protected |
Private |
Public data/ functions in the base class |
Public in the derived class |
Protected in the derived class |
Private in the derived class |
Protected data/ functions in the base class |
Protected in the derived class |
Protected in the derived class |
Private in the derived class |
Private data/fun in the base class |
Not accessible in the derived class |
Not accessible in the derived class |
Not accessible in the derived clas |
Polymorphism
In the base class
virtual void foo();
In the derived class
virtual void foo() override;
Abstract class and pure virtual function
virtual void foo() =0;
Operator overloading
Binary operators
/*1.non-static member function */
complex operator+ (const complex&);
/* 2. non-member function */
friend complex operator* (const
complex&, const complex&);
Unary operators
/*1.non-static member function */
complex operator-();
/*2. non-member function */
friend complex operator~(const complex&);
Inheritance
class A {
// A is a base class }
class B: public A {
// B inherits A.
// B is a derived class}
Dynamic allocation
int *ptr = new int;
delete ptr;
int *ptr = new int[100];
delete [] ptr;
String object
#include<string>
using namespace std;
...
string str1 = "Hello";
string str2 = "World";
Standard C++ Library - <string>
operator[] |
For string a, a[pos]: Return a reference to the character at position pos in the string a. |
operator+= |
Append additional characters at the end of current string. |
operator+ |
Concatenate strings. |
operator< |
Compare string values. For string a and b, a < b: if a is in front of b in dictionary order, return 1, else return 0. |
operator> |
Compare string values. For string a and b, a > b: if a is in back of b in dictionary order, return 1, else return 0. |
operator== |
Compare string values. For string a and b, a == b: if equal, return 1, else return 0; |
operator!= |
Compare string values. For string a and string b, a != b: if not equal, return 1, else return 0; |
compare() |
Compare string. For string a and b, a.compare(b): if equal, return 0. If string a is in front of string b in dictionary order, return -1. If string a is in back of string b in dictionary order, return 1. |
length() |
Return length of string. |
swap() |
Swap string values. |
push_back() |
For string a, a.push_back(c): append character c to the end of the string a, increasing its length by one. |
Standard C++ Library - <sstream>
operator<< |
Retrieves as many characters as possible into the stream. |
operator>> |
Extracts as many characters as possible from the stream. |
str |
Returns a string object with a copy of the current contents of the stream. |
For example(1):
stringstream ss{“Hello”}; // constructor
string str = ss.str(); // a = “Hello”
For example(2):
stringstream ss;
ss<<”75”;
ss<<”76”;
int num;
ss>>num; // num = 7576
Input
Output
Sample Input Download
Sample Output Download
Tags
Discuss
Description
Create a class Matrix to represent an N * N matrix.
Provide public member functions that perform or derive:
1) Matrix(const Matrix &); // copy constructor
2) Matrix &clockwise90();
Rotate a Matrix by 90° clockwise.
NOTE:
If a is a Matrix, the result of a.clockwise90() should be stored back into a. Remember to return the object itself for the use of operation concatenation, such as a.clockwise90().clockwise90(). (You can refer to member function “Matrix &operator=” in function.h.)
3) Overload the stream extraction operator (>>) to read in the matrix elements.
4) Overload the stream insertion operator (<<) to print the content of the matrix row by row.
Note that all of the integers in the same line are separated by a space, and there is a new line character at the end of each line.
Note:
1. This problem involves three files.
- function.h: Class definition of Matrix.
- function.cpp: Member-function definitions of Matrix.
- main.cpp: A driver program to test your class implementation.
You will be provided with function.h and main.cpp, and asked to implement
2. For OJ submission:
Step 1. Include function.h into function.cpp and then implement your function.cpp. (You don’t need to modify function.h and main.cpp)
Step 2. Submit the code of function.cpp into submission block.
Step 3. Check the results and debug your program if necessary.
Input
The first line has an integer N (1<=N<=50), which means the size of the matrix. The total number of elements in the matrix is thus N * N.
For the next N lines specify the elements of the matrix a. All of the integers in the same line are separated by a space.
Output
Your program should print the corresponding results followed by a new line character.
Sample Input Download
Sample Output Download
Partial Judge Code
12261.cppPartial Judge Header
12261.hTags
Discuss
Description
- Mid2 exam accounts for 15% of your semester grade.
- This exam contains three problems. In order to reduce the possibility of traffic jam, the test cases of some problems are divided into several parts.
- The grade of your mid2 exam is computed as follows:
Let P1 be 12261, P2 be 13910-13912, P3 be 13914-13916.
Grade = Score(P1) + Score(P2) * 0.5 + Score(P3) * 0.5,
where Score(Pi) is the number of testcases you earned for problem Pi. - There are totally 15 points.
- {12261} --> {13910-13912} --> {13914-13916}
Input
Output
Sample Input Download
Sample Output Download
Tags
Discuss
Description
This problem is based on "13891 - Polymorphic naming convention conversion".
In this problem, we consider an Integrated Development Environment (IDE) that supports naming convention conversion. We assume that the snake case is the default naming convention:
- snake case is a naming convention where words are separated by underscores (“_”). For example, my_variable_name or my_function_name are examples of the snake case.
The IDE can convert to a different naming convention according to a programmer’s preference. The IDE can also revert back to the default naming convention for the integration among multiple program files.
Branny, an I2P student, after learning the idea of naming conventions, has also created her own two new naming conventions:
- castle case is a naming convention where words are separated by underscores (“_”), and each word has its odd index letters be uppercase and even index letters be lowercase (1-based). For example, My_VaRiAbLe_NaMe or My_FuNcTiOn_NaMe are examples of the castle case.
- number case is a naming convention where words are separated by incremented numbers, which specify the counts of words. For example, my1variable2name or my1function2name are examples of the number case. The numbers would start at 1.
In this problem, we have the abstract base class ‘Case’ as an interface, which contains two pure virtual member functions:
- convert: convert to the desired naming convention;
- revert: revert back to the default naming convention, i.e., the snake case.
#include <string>
using namespace std;
class Case{
protected:
bool converted;
string name;
public:
virtual void convert() = 0;
virtual void revert() = 0;
virtual ~Case(){}
Case(string s): converted(false), name(s){}
void show(ostream &os){
os << name;
}
bool is_converted() const{
return converted;
}
};
class CastleCase: public Case{
public:
CastleCase(string s): Case(s){}
void convert();
void revert();
};
class NumberCase: public Case{
public:
NumberCase(string s): Case(s){}
void convert();
void revert();
};
You are asked to implement the two derived classes ‘CastleCase’ and ‘NumberCase’ by overriding the two pairs of virtual functions, convert and revert, to support the conversion for Branny's preferred naming conventions. :)
Input
The first line contains a string, which can be ‘castle’, ‘number’ or ‘both’.
The second line contains only lowercase letters and underscores, representing the variable name with the snake case.
Constraints:
Testcases 1~3: The first line would be ‘castle’.
Testcases 4~6: The first line would be ‘number’.
Testcases 7~10: The first line would be ‘both’.
Output
The Castle case and the Number case for the input string.
If the first line of input is ‘castle’, print only the castle case.
If the first line of input is ‘number’, print only the number case.
Otherwise, print both of them.
Please refer to the sample output for details.
Sample Input Download
Sample Output Download
Partial Judge Code
13910.cppPartial Judge Header
13910.hTags
Discuss
Description
You are going to broadcast a football game.
The field of this game is length of \(n\), width of \(m\), and there are \(p\) players who's ready to joint this game.
During the game, there would be some events which you need to handle.
Type 1: A player numbered \(player_id\) has catched the ball.
Type 2: The player who's having the ball has kicked it along the direction of (\(dx\), \(dy\)). The ball would stop moving if it has hit the boundary of this field (\(x = 0\), \(x = n-1\), \(y = 0\), \(y = m-1\)), or any player on its way, except the players who was standing at its initial position.
Type 3: The player who's having the ball has kicked it along the direction of (\(dx\), \(dy\)). The ball would stop moving if it has hit any player on its way, except the players who was standing at its initial position. Even if the ball has hit the boundary of the field, it'll keep moing until it cannot move in each direction. (Check the figure below for better understanding)
Type 4: The player who's having the ball has kicked it along the direction of (\(dx\), \(dy\)). The ball would stop moving if it has hit any player on its way, except the players who was standing at its initial position. If the ball has hit the boundary of the field, it'll rebound once and then follow the mechanism as ball in Type 3. (Check the figure below for better understanding)
Type 5: You have to report the current state of this game.
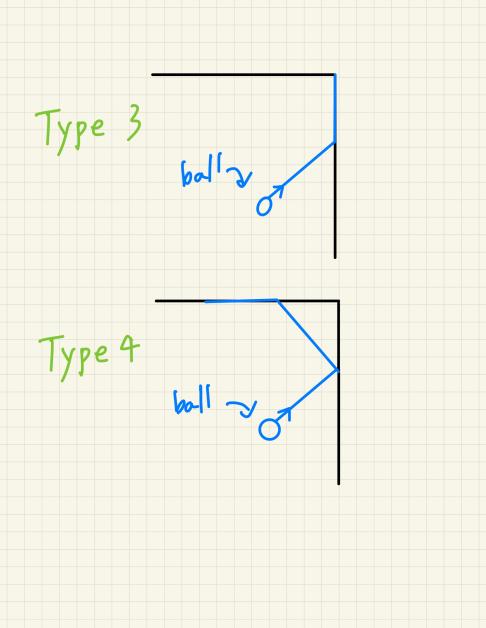
In the example of type 3 above, the following events have happened. 1. The ball has hit the boundary of x = n-1, dx becomes 0. 2. The ball has hit the boundary of y = 0, dy becomes 0. 3. dx = 0 and dy = 0, the ball had stop moving.
In the example of type 4 above, the following events have happened. 1. The ball has hit the boundary of x = n-1, dx *= -1. 2. The ball has hit the boundary of y = 0, dy becomes 0. 3. The ball is flying along its direction...
In this problem, event except type 2, 3, 4 has been implemented for you. You have to implement the function handleBallKicked for type 2, 3, function handleBallKickedRebounce for type 4.